Add structure for container and shapes (#1)
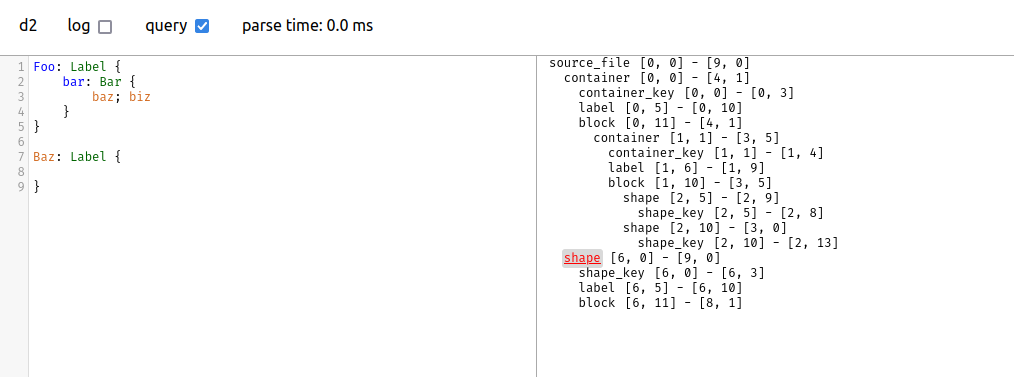 Co-authored-by: Dmitriy Pleshevskiy <dmitriy@ideascup.me> Reviewed-on: #1
This commit is contained in:
parent
a5758ca249
commit
be8db7ca48
13 changed files with 1296 additions and 1238 deletions
2
.gitignore
vendored
2
.gitignore
vendored
|
@ -2,5 +2,7 @@
|
|||
.envrc
|
||||
.direnv
|
||||
|
||||
# test data
|
||||
test.d2
|
||||
# produced by d2
|
||||
*.svg
|
||||
|
|
272
grammar.js
272
grammar.js
|
@ -1,155 +1,194 @@
|
|||
const spaces = repeat(choice(" ", "\t"));
|
||||
const PREC = {
|
||||
COMMENT: -2,
|
||||
EOL: -1,
|
||||
UNQUOTED_STRING: 0,
|
||||
CONTAINER: 2,
|
||||
CONNECTION: 2,
|
||||
SHAPE: 3,
|
||||
IDENTIFIER: 0,
|
||||
ARROW: 0,
|
||||
ATTRIBUTE: 0,
|
||||
ATTRIBUTE_KEY: 0,
|
||||
};
|
||||
|
||||
module.exports = grammar({
|
||||
name: "d2",
|
||||
|
||||
extras: ($) => [$.line_comment],
|
||||
|
||||
word: ($) => $._word,
|
||||
|
||||
conflicts: ($) => [
|
||||
[$.shape_key],
|
||||
[$._shape_path],
|
||||
[$._shape_block],
|
||||
[$._shape_block_definition],
|
||||
[$._style_attr_block],
|
||||
[$._inner_style_attribute],
|
||||
[$._emptyline],
|
||||
extras: ($) => [
|
||||
/[ \f\t\v\u00a0\u1680\u2000-\u200a\u2028\u2029\u202f\u205f\u3000\ufeff]/,
|
||||
$.line_comment,
|
||||
],
|
||||
|
||||
word: ($) => $._identifier,
|
||||
|
||||
conflicts: ($) => [[$._connection_path, $.container]],
|
||||
|
||||
rules: {
|
||||
source_file: ($) => repeat($._root_definition),
|
||||
|
||||
_root_definition: ($) =>
|
||||
choice(
|
||||
$._emptyline,
|
||||
$._root_attribute,
|
||||
$.connection,
|
||||
$._shape_definition
|
||||
$._eol,
|
||||
seq(
|
||||
choice(
|
||||
alias($._root_attribute, $.attribute),
|
||||
$.shape,
|
||||
$.container,
|
||||
$.connection
|
||||
),
|
||||
$._end
|
||||
)
|
||||
),
|
||||
|
||||
// connections
|
||||
|
||||
connection: ($) =>
|
||||
seq(
|
||||
$._shape_path,
|
||||
repeat1(seq($._arrow, $._shape_path)),
|
||||
optional(
|
||||
choice(seq($.dot, $._connection_attribute), seq($._colon, $.label))
|
||||
),
|
||||
$._end
|
||||
$._connection_path,
|
||||
repeat1(seq($.arrow, $._connection_path)),
|
||||
optional(seq($._colon, $.label))
|
||||
),
|
||||
|
||||
_shape_definition: ($) =>
|
||||
_connection_path: ($) =>
|
||||
seq(
|
||||
$._shape_path,
|
||||
optional(
|
||||
choice(
|
||||
seq($.dot, $._shape_attribute),
|
||||
seq(
|
||||
optional(seq($._colon, optional(seq(spaces, $.label)))),
|
||||
optional(alias($._shape_block, $.block))
|
||||
)
|
||||
)
|
||||
repeat(
|
||||
prec(PREC.CONNECTION, seq(alias($.shape_key, $.container_key), $.dot))
|
||||
),
|
||||
$._end
|
||||
),
|
||||
|
||||
_shape_path: ($) =>
|
||||
seq(
|
||||
spaces,
|
||||
repeat(seq(alias($.shape_key, $.container_key), spaces, $.dot, spaces)),
|
||||
$.shape_key
|
||||
),
|
||||
|
||||
shape_key: ($) =>
|
||||
choice(
|
||||
$.string,
|
||||
// containers
|
||||
|
||||
container: ($) =>
|
||||
prec(
|
||||
PREC.CONTAINER,
|
||||
seq(
|
||||
optional($._dash),
|
||||
alias($.shape_key, $.container_key),
|
||||
choice(
|
||||
$._word,
|
||||
repeat1(seq($._word, choice(repeat1(" "), $._dash), $._word))
|
||||
),
|
||||
optional($._dash)
|
||||
seq($.dot, choice($.shape, $.container)),
|
||||
seq(
|
||||
seq(
|
||||
optional(seq($._colon, optional($.label))),
|
||||
optional(seq(alias($._container_block, $.block)))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
),
|
||||
|
||||
_dash: ($) => token.immediate("-"),
|
||||
_container_block: ($) =>
|
||||
seq("{", repeat($._container_block_definition), "}"),
|
||||
|
||||
label: ($) => choice($.string, $._unquoted_string),
|
||||
_container_block_definition: ($) =>
|
||||
choice(
|
||||
$._eol,
|
||||
seq(
|
||||
choice($.shape, $.container, $.connection, $._shape_attribute),
|
||||
$._end
|
||||
)
|
||||
),
|
||||
|
||||
attr_value: ($) => seq(spaces, choice($.string, $._unquoted_string)),
|
||||
// shapes
|
||||
|
||||
shape: ($) =>
|
||||
prec(
|
||||
PREC.SHAPE,
|
||||
seq(
|
||||
$.shape_key,
|
||||
optional(
|
||||
choice(
|
||||
seq($.dot, alias($._style_attribute, $.attribute)),
|
||||
seq(optional(seq($._colon, optional($.label))))
|
||||
)
|
||||
)
|
||||
)
|
||||
),
|
||||
|
||||
shape_key: ($) => choice($.string, seq($._identifier, optional($._dash))),
|
||||
|
||||
_identifier: ($) =>
|
||||
token(prec(PREC.IDENTIFIER, /\-?([\w\d]+|([\w\d]+( +|\-)[\w\d]+)+)/)),
|
||||
|
||||
// attributes
|
||||
|
||||
_root_attribute: ($) =>
|
||||
seq(alias($._root_attr_key, $.attr_key), $._colon, $.attr_value),
|
||||
|
||||
_root_attr_key: ($) =>
|
||||
choice(
|
||||
seq(
|
||||
alias($._root_attr_key, $.attr_key),
|
||||
$._colon,
|
||||
$.attr_value,
|
||||
$._end
|
||||
),
|
||||
alias(seq($._shape_attribute, $._end), $.invalid)
|
||||
"direction",
|
||||
// reserved but doesn't affected for root
|
||||
alias(
|
||||
choice(
|
||||
"shape",
|
||||
"label",
|
||||
"constraint",
|
||||
"icon",
|
||||
"style",
|
||||
$._common_style_attr_key,
|
||||
$._text_attr_key
|
||||
),
|
||||
$.reserved
|
||||
)
|
||||
),
|
||||
|
||||
_root_attr_key: ($) => "direction",
|
||||
|
||||
_shape_block: ($) =>
|
||||
seq(
|
||||
spaces,
|
||||
"{",
|
||||
repeat(choice($._emptyline, seq(spaces, $._shape_block_definition))),
|
||||
optional(seq($._shape_block_definition, optional($._end))),
|
||||
spaces,
|
||||
"}"
|
||||
),
|
||||
|
||||
_shape_block_definition: ($) =>
|
||||
choice($.connection, $._shape_definition, $._shape_attribute),
|
||||
|
||||
_shape_attribute: ($) =>
|
||||
choice(
|
||||
seq(alias($._shape_attr_key, $.attr_key), $._colon, $.attr_value),
|
||||
$._style_attribute
|
||||
alias($._base_shape_attribute, $.attribute),
|
||||
alias($._style_attribute, $.attribute)
|
||||
),
|
||||
|
||||
_style_attribute: ($) =>
|
||||
seq(
|
||||
alias("style", $.attr_key),
|
||||
_base_shape_attribute: ($) =>
|
||||
seq(alias($._shape_attr_key, $.attr_key), $._colon, $.attr_value),
|
||||
|
||||
_shape_attr_key: ($) =>
|
||||
prec(
|
||||
PREC.ATTRIBUTE_KEY,
|
||||
choice(
|
||||
seq($.dot, $._inner_style_attribute),
|
||||
seq($._colon, alias($._style_attr_block, $.block))
|
||||
"shape",
|
||||
"label",
|
||||
// sql
|
||||
"constraint",
|
||||
// image
|
||||
"icon",
|
||||
"width",
|
||||
"height"
|
||||
)
|
||||
),
|
||||
|
||||
_style_attr_block: ($) =>
|
||||
_style_attribute: ($) =>
|
||||
prec(
|
||||
PREC.ATTRIBUTE,
|
||||
seq(
|
||||
alias("style", $.attr_key),
|
||||
choice(
|
||||
seq($.dot, alias($._inner_style_attribute, $.attribute)),
|
||||
seq($._colon, alias($._style_attribute_block, $.block))
|
||||
)
|
||||
)
|
||||
),
|
||||
|
||||
_style_attribute_block: ($) =>
|
||||
seq(
|
||||
spaces,
|
||||
"{",
|
||||
spaces,
|
||||
repeat(choice($._emptyline, seq($._inner_style_attribute, $._end))),
|
||||
optional(seq($._inner_style_attribute, optional($._end))),
|
||||
spaces,
|
||||
repeat(
|
||||
choice(
|
||||
$._eol,
|
||||
seq(alias($._inner_style_attribute, $.attribute), $._end)
|
||||
)
|
||||
),
|
||||
"}"
|
||||
),
|
||||
|
||||
_inner_style_attribute: ($) =>
|
||||
seq(spaces, alias($._style_attr_key, $.attr_key), $._colon, $.attr_value),
|
||||
|
||||
_connection_attribute: ($) =>
|
||||
seq(alias($._connection_attr_key, $.attr_key), $._colon, $.attr_value),
|
||||
|
||||
_shape_attr_key: ($) =>
|
||||
choice(
|
||||
"shape",
|
||||
"label",
|
||||
// sql
|
||||
"constraint",
|
||||
// image
|
||||
"icon",
|
||||
"width",
|
||||
"height"
|
||||
prec(
|
||||
PREC.ATTRIBUTE,
|
||||
seq(alias($._style_attr_key, $.attr_key), $._colon, $.attr_value)
|
||||
),
|
||||
|
||||
_style_attr_key: ($) =>
|
||||
_style_attr_key: ($) => choice($._common_style_attr_key, "3d"),
|
||||
|
||||
_common_style_attr_key: ($) =>
|
||||
choice(
|
||||
"opacity",
|
||||
"fill",
|
||||
|
@ -161,7 +200,6 @@ module.exports = grammar({
|
|||
"shadow",
|
||||
"multiple",
|
||||
"animated",
|
||||
"3d",
|
||||
"link"
|
||||
),
|
||||
|
||||
|
@ -169,15 +207,24 @@ module.exports = grammar({
|
|||
|
||||
_connection_attr_key: ($) => choice("source-arrowhead", "target-arrowhead"),
|
||||
|
||||
_colon: ($) => seq(spaces, ":"),
|
||||
//
|
||||
|
||||
_arrow: ($) => seq(spaces, $.arrow),
|
||||
label: ($) => choice($.string, $._unquoted_string),
|
||||
|
||||
arrow: ($) => choice(/-+>/, /--+/, /<-+/, /<-+>/),
|
||||
attr_value: ($) => seq(choice($.string, $._unquoted_string)),
|
||||
|
||||
dot: ($) => token.immediate("."),
|
||||
// --------------------------------------------
|
||||
|
||||
_unquoted_string: ($) => token.immediate(/[^'"`\n;{}]+/),
|
||||
_dash: ($) => token.immediate("-"),
|
||||
|
||||
_colon: ($) => seq(":"),
|
||||
|
||||
arrow: ($) => token(prec(PREC.ARROW, choice(/-+>/, /--+/, /<-+/, /<-+>/))),
|
||||
|
||||
dot: ($) => token("."),
|
||||
|
||||
_unquoted_string: ($) =>
|
||||
token(prec(PREC.UNQUOTED_STRING, /[\w\-?!]([^'"`\n;{}]*[\w\-?!])?/)),
|
||||
|
||||
string: ($) =>
|
||||
choice(
|
||||
|
@ -186,12 +233,9 @@ module.exports = grammar({
|
|||
seq("`", repeat(token.immediate(/[^`\n]+/)), "`")
|
||||
),
|
||||
|
||||
line_comment: ($) => token(prec(-2, seq("#", /.*/))),
|
||||
line_comment: ($) => token(prec(PREC.COMMENT, seq("#", /.*/))),
|
||||
|
||||
_word: ($) => /[\w\d]+/,
|
||||
|
||||
_emptyline: ($) => seq(spaces, $._eof),
|
||||
_eof: ($) => choice("\n", "\0"),
|
||||
_end: ($) => seq(spaces, choice(";", $._eof)),
|
||||
_eol: ($) => token(prec(PREC.EOL, "\n")),
|
||||
_end: ($) => seq(choice(";", $._eol)),
|
||||
},
|
||||
});
|
||||
|
|
|
@ -1,13 +1,9 @@
|
|||
; Special (treesitter don't overwrite)
|
||||
;-------------------------------------------------------------------------------
|
||||
|
||||
(ERROR) @error
|
||||
(invalid (_) @error)
|
||||
;-------------------------------------------------------------------------------
|
||||
|
||||
(container_key) @constant
|
||||
(shape_key) @variable
|
||||
(attr_key) @property
|
||||
(reserved) @error
|
||||
|
||||
; Literals
|
||||
;-------------------------------------------------------------------------------
|
||||
|
@ -42,4 +38,3 @@
|
|||
;-------------------------------------------------------------------------------
|
||||
|
||||
(ERROR) @error
|
||||
(invalid (_) @error)
|
||||
|
|
1541
src/grammar.json
1541
src/grammar.json
File diff suppressed because it is too large
Load diff
|
@ -1,13 +1,18 @@
|
|||
[
|
||||
{
|
||||
"type": "arrow",
|
||||
"named": true,
|
||||
"fields": {}
|
||||
},
|
||||
{
|
||||
"type": "attr_key",
|
||||
"named": true,
|
||||
"fields": {}
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": false,
|
||||
"required": false,
|
||||
"types": [
|
||||
{
|
||||
"type": "reserved",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "attr_value",
|
||||
|
@ -25,12 +30,12 @@
|
|||
}
|
||||
},
|
||||
{
|
||||
"type": "block",
|
||||
"type": "attribute",
|
||||
"named": true,
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": true,
|
||||
"required": false,
|
||||
"required": true,
|
||||
"types": [
|
||||
{
|
||||
"type": "attr_key",
|
||||
|
@ -40,28 +45,43 @@
|
|||
"type": "attr_value",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "attribute",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "block",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "block",
|
||||
"named": true,
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": true,
|
||||
"required": false,
|
||||
"types": [
|
||||
{
|
||||
"type": "attribute",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "connection",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "container_key",
|
||||
"type": "container",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "label",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"type": "shape",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
|
@ -80,11 +100,38 @@
|
|||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "attr_key",
|
||||
"type": "container_key",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "attr_value",
|
||||
"type": "dot",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "label",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "container",
|
||||
"named": true,
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": true,
|
||||
"required": true,
|
||||
"types": [
|
||||
{
|
||||
"type": "block",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "container",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
|
@ -100,7 +147,7 @@
|
|||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"type": "shape",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
|
@ -121,33 +168,6 @@
|
|||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "invalid",
|
||||
"named": true,
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": true,
|
||||
"required": false,
|
||||
"types": [
|
||||
{
|
||||
"type": "attr_key",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "attr_value",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "block",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "label",
|
||||
"named": true,
|
||||
|
@ -163,6 +183,38 @@
|
|||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "reserved",
|
||||
"named": true,
|
||||
"fields": {}
|
||||
},
|
||||
{
|
||||
"type": "shape",
|
||||
"named": true,
|
||||
"fields": {},
|
||||
"children": {
|
||||
"multiple": true,
|
||||
"required": true,
|
||||
"types": [
|
||||
{
|
||||
"type": "attribute",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "label",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
}
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"named": true,
|
||||
|
@ -187,15 +239,7 @@
|
|||
"required": false,
|
||||
"types": [
|
||||
{
|
||||
"type": "attr_key",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "attr_value",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "block",
|
||||
"type": "attribute",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
|
@ -203,23 +247,11 @@
|
|||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "container_key",
|
||||
"type": "container",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "invalid",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "label",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "shape_key",
|
||||
"type": "shape",
|
||||
"named": true
|
||||
}
|
||||
]
|
||||
|
@ -230,22 +262,6 @@
|
|||
"named": true,
|
||||
"fields": {}
|
||||
},
|
||||
{
|
||||
"type": "\u0000",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "\t",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "\n",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": " ",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "\"",
|
||||
"named": false
|
||||
|
@ -274,6 +290,10 @@
|
|||
"type": "animated",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "arrow",
|
||||
"named": true
|
||||
},
|
||||
{
|
||||
"type": "border-radius",
|
||||
"named": false
|
||||
|
@ -282,6 +302,10 @@
|
|||
"type": "constraint",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "direction",
|
||||
"named": false
|
||||
},
|
||||
{
|
||||
"type": "dot",
|
||||
"named": true
|
||||
|
|
BIN
src/parser.c
BIN
src/parser.c
Binary file not shown.
|
@ -3,59 +3,75 @@ Root attribute
|
|||
================================================================================
|
||||
direction: value
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(attr_key) (attr_value)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Block shape attribute
|
||||
================================================================================
|
||||
foo: {
|
||||
shape: oval
|
||||
|
||||
foo.bar.baz: {
|
||||
shape: oval
|
||||
}
|
||||
}
|
||||
shape: oval
|
||||
label: ten
|
||||
constraint: utehu
|
||||
icon: huell
|
||||
opacity: 1
|
||||
fill: red
|
||||
stroke: red
|
||||
stroke-width: 5
|
||||
stroke-dash: 4
|
||||
border-radius: 1
|
||||
font-color: red
|
||||
shadow: true
|
||||
multiple: true
|
||||
animated: true
|
||||
link: https://to
|
||||
near: abc
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(block
|
||||
(attr_key) (attr_value)
|
||||
(container_key) (dot) (container_key) (dot) (shape_key)
|
||||
(block
|
||||
(attr_key) (attr_value)
|
||||
)
|
||||
)
|
||||
)
|
||||
(attribute (attr_key) (attr_value))
|
||||
|
||||
|
||||
================================================================================
|
||||
Inline shape attribute
|
||||
================================================================================
|
||||
foo.shape: oval
|
||||
foo.bar.baz.shape: oval
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (dot) (attr_key) (attr_value)
|
||||
(container_key) (dot) (container_key) (dot) (shape_key) (dot) (attr_key) (attr_value)
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
(attribute (attr_key (reserved)) (attr_value))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Inline style attribute
|
||||
Style attribute
|
||||
================================================================================
|
||||
foo.style.opacity: 5
|
||||
foo.style.fill: red
|
||||
foo.style.stroke: red
|
||||
foo.style.stroke-width: 5
|
||||
foo.style.stroke-dash: 4
|
||||
foo.style.border-radius: 1
|
||||
foo.style.font-color: red
|
||||
foo.style.shadow: true
|
||||
foo.style.multiple: true
|
||||
foo.style.animated: true
|
||||
foo.style.link: https://to
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (dot) (attr_key) (dot) (attr_key) (attr_value)
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
(shape (shape_key) (dot) (attribute (attr_key) (dot) (attribute (attr_key) (attr_value))))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -63,48 +79,150 @@ Block style attributes
|
|||
================================================================================
|
||||
foo.style: {
|
||||
opacity: 5
|
||||
|
||||
fill: red;
|
||||
fill: red
|
||||
stroke: red
|
||||
stroke-width: 5
|
||||
stroke-dash: 4
|
||||
border-radius: 1
|
||||
font-color: red
|
||||
shadow: true
|
||||
multiple: true
|
||||
animated: true
|
||||
link: https://to
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (dot) (attr_key)
|
||||
(block
|
||||
(attr_key) (attr_value)
|
||||
(attr_key) (attr_value)
|
||||
(shape
|
||||
(shape_key) (dot)
|
||||
(attribute
|
||||
(attr_key)
|
||||
(block
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Inline block style attributes
|
||||
Container attributes
|
||||
================================================================================
|
||||
foo.style: { opacity: 5; fill: red; }
|
||||
foo.style: { opacity: 5 }
|
||||
foo: {
|
||||
shape: oval
|
||||
label: Baz
|
||||
constraint: primary-key
|
||||
icon: pathto
|
||||
width: 100
|
||||
height: 200
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (dot) (attr_key)
|
||||
(block
|
||||
(attr_key) (attr_value)
|
||||
(attr_key) (attr_value)
|
||||
)
|
||||
(shape_key) (dot) (attr_key)
|
||||
(block
|
||||
(attr_key) (attr_value)
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Color in string
|
||||
Container style attributes
|
||||
================================================================================
|
||||
foo.style.fill: "#ffffff";
|
||||
foo: {
|
||||
style.opacity: 5
|
||||
style.fill: red
|
||||
style.stroke: red
|
||||
style.stroke-width: 5
|
||||
style.stroke-dash: 4
|
||||
style.border-radius: 1
|
||||
style.font-color: red
|
||||
style.shadow: true
|
||||
style.multiple: true
|
||||
style.animated: true
|
||||
style.link: https://to
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (dot) (attr_key) (dot) (attr_key) (attr_value (string))
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
(attribute (attr_key) (dot) (attribute (attr_key) (attr_value)))
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Block style attributes inside a container
|
||||
================================================================================
|
||||
foo: {
|
||||
style: {
|
||||
opacity: 5
|
||||
fill: red
|
||||
stroke: red
|
||||
stroke-width: 5
|
||||
stroke-dash: 4
|
||||
border-radius: 1
|
||||
font-color: red
|
||||
shadow: true
|
||||
multiple: true
|
||||
animated: true
|
||||
link: https://to
|
||||
}
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(attribute
|
||||
(attr_key)
|
||||
(block
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
(attribute (attr_key) (attr_value))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
|
|
|
@ -196,3 +196,29 @@ foo.biz.baz -> bar.baz.biz: Label
|
|||
(label)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Connection of shapes inside a block of container
|
||||
================================================================================
|
||||
foo.baz: {
|
||||
foo -> biz: Label
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container
|
||||
(container_key) (dot)
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(connection
|
||||
(shape_key)
|
||||
(arrow)
|
||||
(shape_key)
|
||||
(label)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
|
|
@ -2,22 +2,36 @@
|
|||
Declare a shape inside a container
|
||||
================================================================================
|
||||
foo.baz
|
||||
foo.bar.biz
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container_key) (dot) (shape_key)
|
||||
(container
|
||||
(container_key) (dot)
|
||||
(shape (shape_key))
|
||||
)
|
||||
(container
|
||||
(container_key) (dot)
|
||||
(container
|
||||
(container_key) (dot)
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Use quoted string as a shape key
|
||||
Use quoted string as keys
|
||||
================================================================================
|
||||
'foo'.'baz'
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container_key (string)) (dot) (shape_key (string))
|
||||
(container
|
||||
(container_key (string)) (dot)
|
||||
(shape (shape_key (string)))
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -35,20 +49,26 @@ foo: {
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Declare labaled container inside a labeled container using block
|
||||
Declare labeled container inside a labeled container using block
|
||||
================================================================================
|
||||
|
||||
foo: Foo {
|
||||
|
@ -62,13 +82,22 @@ foo: Foo {
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(shape (shape_key) (label))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
@ -87,11 +116,111 @@ foo: {
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
|
||||
================================================================================
|
||||
Declare a container with complex keys
|
||||
================================================================================
|
||||
|
||||
Foo biz bar: {
|
||||
bar biz baz: {
|
||||
-biz-baz-Baz-: {
|
||||
Helo world
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(block
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Declare a container with complex keys and labels
|
||||
================================================================================
|
||||
|
||||
Foo biz bar: Biz biz Bar {
|
||||
bar biz baz: baz baz biz {
|
||||
-biz-baz-Baz-: Biz buz Baz {
|
||||
Helo world
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Declare shapes sparsely in a container
|
||||
================================================================================
|
||||
|
||||
Foo: Baz {
|
||||
|
||||
biz
|
||||
|
||||
baz
|
||||
|
||||
bar
|
||||
|
||||
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(container
|
||||
(container_key)
|
||||
(label)
|
||||
(block
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
)
|
||||
)
|
||||
)
|
||||
|
|
|
@ -7,8 +7,8 @@ bar
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -20,8 +20,8 @@ Complex shape key
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -32,7 +32,7 @@ Use quoted string as a shape key
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key (string))
|
||||
(shape (shape_key (string)))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -43,9 +43,9 @@ a;b;c
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(shape_key)
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
(shape (shape_key))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -57,9 +57,9 @@ a: Foo Bar; b: Biz Baz
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (label)
|
||||
(shape_key) (label)
|
||||
(shape_key) (label)
|
||||
(shape (shape_key) (label))
|
||||
(shape (shape_key) (label))
|
||||
(shape (shape_key) (label))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
|
@ -73,62 +73,19 @@ bar : Foo Bar; baz
|
|||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(shape_key) (label)
|
||||
(shape_key)
|
||||
(shape (shape_key))
|
||||
(shape (shape_key) (label))
|
||||
(shape (shape_key))
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Shape block
|
||||
Use quoted string as shape key and label
|
||||
================================================================================
|
||||
|
||||
foo: {
|
||||
bar: {
|
||||
baz: {
|
||||
biz
|
||||
}
|
||||
}
|
||||
}
|
||||
'foo': "Label"
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
(block
|
||||
(shape_key)
|
||||
)
|
||||
)
|
||||
)
|
||||
)
|
||||
|
||||
================================================================================
|
||||
Aliased shape block
|
||||
================================================================================
|
||||
|
||||
foo: Foo {
|
||||
bar: Bar {
|
||||
baz: Baz {
|
||||
biz: Biz
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
--------------------------------------------------------------------------------
|
||||
|
||||
(source_file
|
||||
(shape_key) (label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
(block
|
||||
(shape_key) (label)
|
||||
)
|
||||
)
|
||||
)
|
||||
(shape (shape_key (string)) (label (string)))
|
||||
)
|
||||
|
||||
|
|
|
@ -6,7 +6,7 @@ direction: right;
|
|||
|
||||
shape: oval
|
||||
# <- error
|
||||
# ^ error
|
||||
# ^ string
|
||||
|
||||
baaaz.style: {
|
||||
# <- variable
|
|
@ -15,7 +15,7 @@ foo: Foo Bar
|
|||
# ^ string
|
||||
|
||||
foo: Foo Bar {
|
||||
# <- variable
|
||||
# <- constant
|
||||
# ^ string
|
||||
# ^ punctuation.bracket
|
||||
|
||||
|
|
Binary file not shown.
Loading…
Reference in a new issue